Within the programming world, Python stands aside from the remainder of the coding languages due to its readability and ease of use qualities. Nevertheless, it’s usually criticized resulting from its efficiency. However, with the best techniques, efficiency optimization in Python can simply be performed. This text digs deep into a number of methods to enhance Python code effectivity, from greedy its efficiency traits to leveraging concurrency and superior optimization strategies. Whether or not you’re a naive or an completed developer, these particulars will assist you write faster and extra environment friendly Python functions. With that over, now it’s time to uncover:
What’s Python?
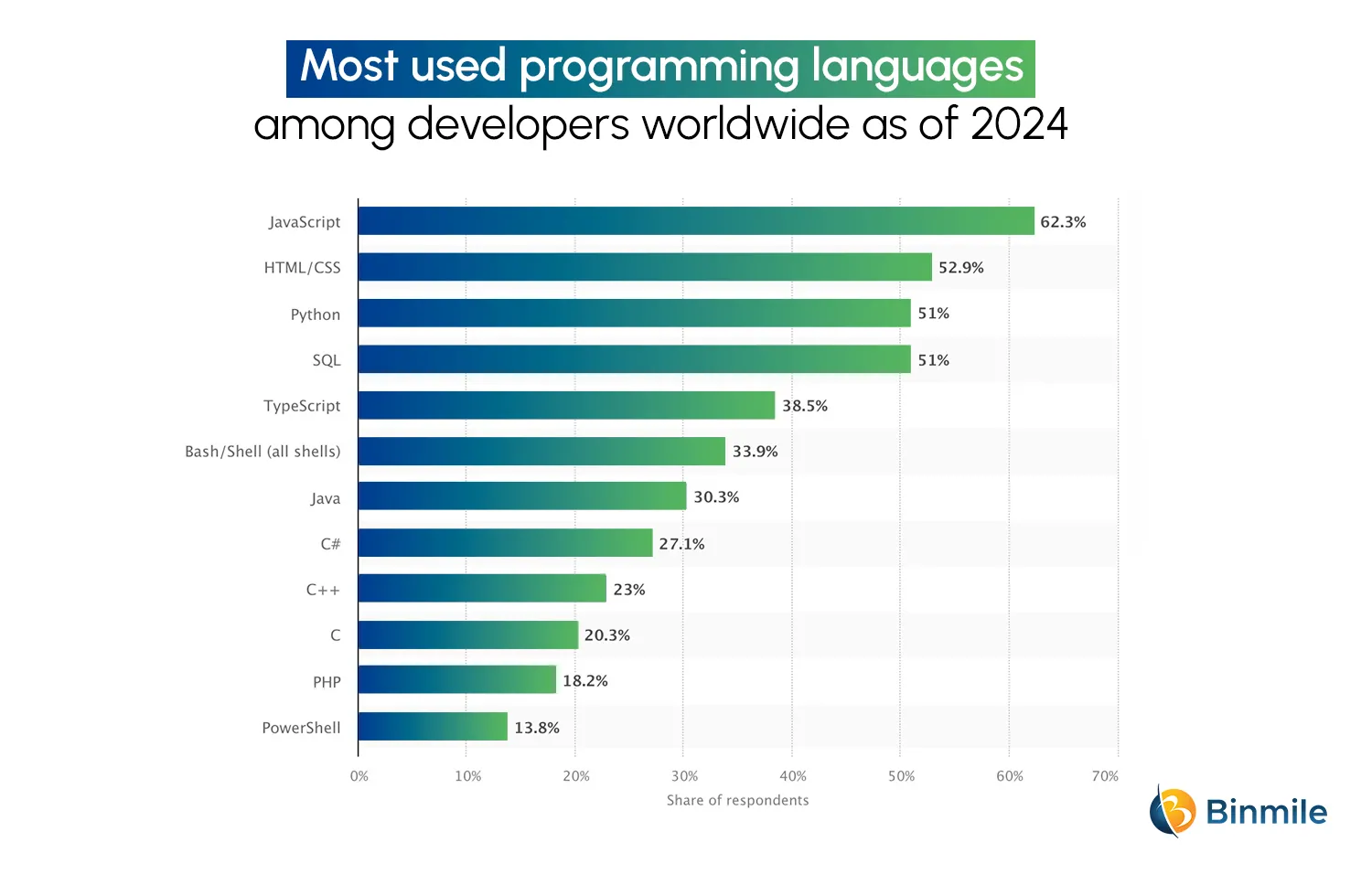
Python is a well-known programming language, used for internet app improvement, knowledge science, and lots of different functions. It’s a higher-level language in comparison with C++ and Java, which implies it abstracts pc particulars for the developer, together with:
- Reminiscence administration
- Pointers
- Threads
These abstractions improve coder productiveness and make Python a breeze to be taught and use. This additionally implies that the directions written by programmers want extra sources to execute as machine code. Additionally, Python applications are slower in comparison with lower-level languages. The excellent news is that it’s possible to hold out efficiency optimization in Python and simply improve its efficiency. That’s the reason, 51% of builders use Python for his or her internet/app improvement wants, as per a worldwide knowledge and enterprise intelligence platform on the market.
What’s Python Efficiency Optimization?
As , Python is comparatively gradual compared to different programming languages, the applications written in C++, Node.js, and Go can execute 30-40x faster than Python applications. There are a number of causes, comprising the truth that Python is an interpreted language (code requires to be compiled all through runtime), dynamically typed, and operates on a single thread.
Python Efficiency Optimization is the method of tweaking the efficiency of Python applications, whatever the vital disadvantages of the know-how. We’ll talk about some frequent methods for Python optimization on this content material, together with profiling, caching, reminiscence optimization, and lifeless code removing.
Most Efficient Strategies for Efficiency Optimization in Python
1. Implementing Caching and Memoization
Caching and Memoization are sturdy methods for Python code efficiency optimization. By storing the outcomes of costly operate calls, these strategies remove the necessity for redundant computations, saving a considerable period of time and sources. When a operate known as with the identical inputs, the precomputed outcomes enter the image and are instantly retrieved from the cache, leading to quicker execution. The steps concerned on this course of are:
- Acknowledge features with costly computations or repeated calls
- Implement caching with the assistance of functools.Iru_cache decorator in Python
- Leverage Memoization libraries or personalized options for extra difficult eventualities
Caching and Memoization can closely scale back the execution time of Python code, particularly in apps with intensive knowledge processing or difficult calculations. It’s essential to consider the steadiness between reminiscence utilization and velocity, as caching consumes further reminiscence to maintain the outcomes.
Appropriate implementation of Caching and Memoization can result in a exceptional enhancement in utility responsiveness and effectivity. As per a number one Python improvement firm, it’s a way that gels effectively with Python’s design philosophy of readability and ease, enabling programmers to write down high-performing code with out degrading code high quality.
2. Utilizing Cython for Efficiency Beneficial properties
Cython emerges as a stable software for builders trying to enhance the efficiency of Python for internet improvement initiatives, particularly when concerned in mathematical computing. By letting Python code be compiled into C, Cython can sharply decrease execution time for computationally intensive duties.
To correctly use Cython, one ought to discover the essential components of the applying which might be bottlenecks in efficiency. After discovering these areas, coders can rewrite them in Cython, benefiting from C-level velocity with out compromising on the readability and ease of Python.
This specific software not solely hastens the execution but additionally affords clean integration with C or C++ libraries, permitting programmers to achieve entry to an surroundings of improved, low-level code. Along with that, one can use this software together with different Python optimization suggestions, similar to profiling, caching, and algorithmic enhancements. By combining these strategies, coders can accomplish a radical optimization of their Python code.
3. Reminiscence Optimization with Slots
Python’s slots mechanism affords a way to keep away from the normal dictionary-centric storage for object attributes. By defining slots in a category, you give directions to Python to allot area for a set set of attributes, translating into vital reminiscence financial savings and faster attribute entry. That is significantly useful in conditions the place hundreds of thousands of cases of a category are developed.
- Reminiscence Effectivity: Slots retailer properties in a bite-sized tuple-like construction that’s extra memory-efficient in comparison with a dictionary.
- Higher Code Readability: A transparent definition of slots can improve code readability and maintainability.
- Attribute Restriction: Slots stop the dynamic improvement of recent attributes, fostering higher encapsulation.
- Quicker Attribute Entry: By eliminating dictionary searches, slots can speed up attribute entry.
Have in mind, slots will not be a treatment for all performance-related troubles, however they’re a useful gizmo for enhancing reminiscence utilization in particular conditions. It’s vital to understand when their use is apt and how you can implement them correctly to leverage the benefits they provide.
4. Interning Strings for Effectivity
In case you might be unversed, interning a string is a technique of storing only one copy of each distinctive string. You can even make the Python interpreter reuse strings by altering the code to make sure string interning. Once we develop a string object, the Python interpreter often determines whether or not or not the string ought to be cached. In keeping with a digital transformation firm, the interpreter’s underlying qualities come out in particular conditions, like when processing identifiers.
Declaring a string with a reputation that begins with a letter or an underscore and accommodates solely or mixture of letters, underscores, and integers makes Python intern the string and create a hash for it. Python accommodates wads of inside code that make the most of dictionaries, inflicting it to carry out a myriad of identifier searches. Resultantly, interning the identification strings expedites the entire course of, considerably contributing to Python efficiency optimization. Merely perceive, Python retains all identifiers in a desk and develops distinctive keys (hashes) for each merchandise for future searches. This optimization takes place all through the compilation course of. It additionally includes the intertwining of string literals that appear as if identifiers. Consequently, it’s a helpful characteristic in Python you can take advantage of. Such a performance may help in processing appreciable textual content mining or analytics functions as a result of they compel frequent lookups and message flip-flopping for bookkeeping.
The auto-interning in Python doesn’t have strings learn from a file or acquired by way of community communication. As an alternative, you possibly can assign this work to the intern() operate accountable for managing such circumstances.
5. Eradicating Useless Code
Useless code makes use of processing energy and makes Python optimizations and code output a bit sluggish. Due to this fact, customized internet improvement companies supplier counsel reviewing your Python code ceaselessly and hunting down pointless or redundant code to save lots of reminiscence. Listed below are some confirmed methods to take away unused code:
- Context managers: They come in useful in hiding or unhiding operate code. When a operate is hidden, the reminiscence continues to be unblocked. This may speed up your applications and clear up your code by clearing reminiscence for the code that’s imagined to run.
- Preload processes: If a program takes a very long time to carry out sure operations, you possibly can preload these operations, and have the outcomes prepared when wanted for frequent duties.
- Multiprocessing: Though Python doesn’t help multi-threaded operations, you possibly can execute a number of Python processes concurrently, utilizing further reminiscence banks totally different from current working reminiscence. You possibly can entry reminiscence on further servers to run background processes in an environment friendly method.
Take your Python internet utility to the following degree! Allow us to optimize efficiency for quicker, extra scalable outcomes.
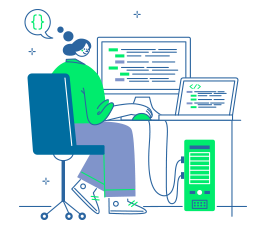
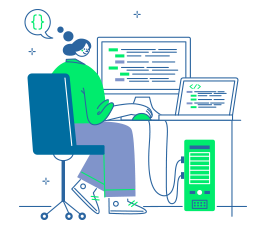
High 4 Instruments for Optimizing Python Code for Efficiency
#1 DeepSource
Deepsource analyses static code in a number of coding languages, together with however not restricted to Python, Javascript, Golang, and so forth. It affords the pliability and the potential to do static evaluation on Python code simply. This specific software creates a Configuration File that’s stored within the repository, and the code is assessed ceaselessly.
A couple of options of DeepSource embody:
- To make sure steady evaluation, make the most of a single-file configuration
- To check protection, combine in CI/CD pipelines, similar to Travis CI
- Code codecs, like Black and AutoPep8, are supported
- Resolutions for frequent points which might be automated
- Every pull goes via a high quality test
Not like different static code evaluation instruments on the market, DeepSource has a low false-positive price and a quick decision time, in terms of efficiency optimization in Python. This makes it a breeze for maintainers to judge framework-related issues by offering them entry to them.
#2 Codacy
Codacy is the following software on this checklist that gives code protection and evaluation reviews for a number of general-purpose programming languages, like Python frameworks revolutionizing internet app improvement. This software solves all kinds of considerations, similar to duplication, complexity, and code protection. It helps programmers keep code high quality and a clear code evaluation.
Codacy has a mess of traits, together with:
- Code evaluation will be automated
- Builders can leverage automated useful resource options
- Examine the standard of code over time
- Analyze and commit each pull request individually
- To keep away from chaos, solely new bugs are thought-about
#3 Checkmarx
Checkmarx is likely one of the finest instruments for state code evaluation and utility safety testing and finest practices. It contains skills, like static app testing, interactive testing, runtime testing, and dependency scanning, that allow swift scanning of supply code and the decision of bugs.
Beneath are some options that grow to be helpful for efficiency optimization in Python:
- Checkmarx SAST is tapped for static evaluation and figuring out safety flaws
- The UI is intuitive and easy to make use of
- Incorporates with steady integration and supply pipelines
- Plugins for a battery of well-known IDEs (Built-in Improvement Setting) can be found
#4 Veracode
Veracode can also be a reputed Python code evaluation software. It not solely examines frequent vulnerabilities and exposures, however it may additionally uncover points utilizing static evaluation, making it simple to report defects and anti-patterns. This software additionally supplies different companies by way of its enterprise service, like interactive and dynamic evaluation.
Listed below are some vital options that help whereas optimizing Python code for efficiency:
- Scanning with SCA (Software program Composition Evaluation) brokers to seek out faults and vulnerabilities
- Preserving PyPi’s libraries and license up to date
- With each scan, it sends a danger rating
- Incorporation with DevOps processes is clean
- To make code high quality checking less complicated, it renders developer instruments, an Utility Programming Interface, and workflow integration
Need assistance optimizing your Python internet app? Our Python improvement companies can improve your utility’s efficiency and velocity massive time.
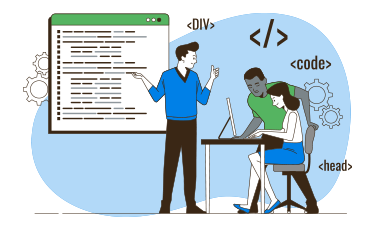
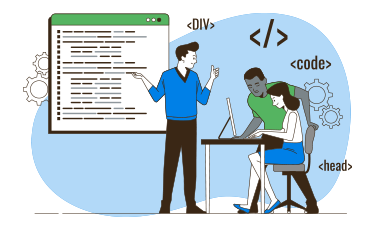
On a Concluding Observe!
We hope you realized one of the best methodology of Python code optimization via this content material. From using built-in features and libraries to perceiving the facility of “slots” for reminiscence effectivity, now we have mentioned the importance of profiling, caching, and algorithmic optimization. Thus, it’s clear that Python builders should apply these optimization strategies ceaselessly to create faster, extra environment friendly, and scalable functions. Should you nonetheless discover it difficult to spice up your code’s efficiency in 2025, it’s a clever determination to attach with service executives of a trusted app improvement firm to optimize your present mission.